Creating a Serverless Application with Node.js and AWS Lambda
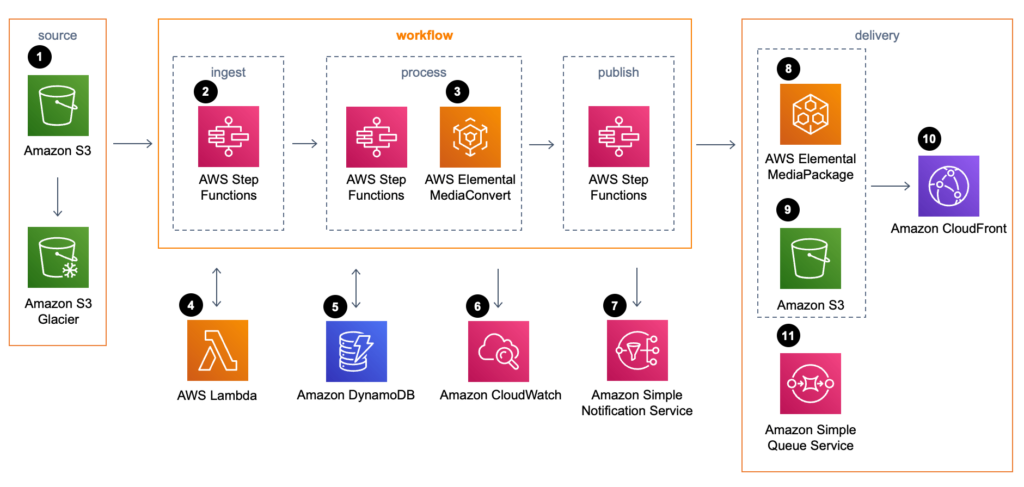
Introduction
Serverless computing has revolutionized the way we develop and deploy web applications. Instead of provisioning and managing servers, developers can focus on writing code for their application logic while cloud providers like Amazon Web Services (AWS) take care of the underlying infrastructure. AWS Lambda is a popular serverless computing platform that allows developers to build and run applications without worrying about servers, and it supports multiple programming languages including Node.js.
In this blog post, we will explore how to create a serverless application with Node.js and AWS Lambda. We will cover the basics of serverless computing, AWS Lambda, and Node.js, and then we will walk through the process of building a serverless application that uses AWS Lambda and other AWS services.
Part 1: Understanding Serverless Computing and AWS Lambda
Before we dive into the details of building a serverless application, let’s take a moment to understand what serverless computing is and how AWS Lambda fits into the picture.
Serverless computing is a cloud computing model that allows developers to build and run applications without worrying about the underlying infrastructure. In traditional server-based computing, developers need to provision and manage servers, operating systems, and other infrastructure components. With serverless computing, the cloud provider takes care of all of these tasks, allowing developers to focus on writing code for their application logic.
AWS Lambda is a popular serverless computing platform that allows developers to build and run applications in response to events or HTTP requests. AWS Lambda functions can be triggered by a wide range of events, including changes to data in Amazon S3 buckets, updates to Amazon DynamoDB tables, and custom events generated by other AWS services.
AWS Lambda supports multiple programming languages, including Node.js, Java, Python, and C#. In this blog post, we will focus on using Node.js to build a serverless application with AWS Lambda.
Part 2: Building a Serverless Application with Node.js and AWS Lambda
To build a serverless application with Node.js and AWS Lambda, we will need to perform the following steps:
- Set up an AWS account and create an IAM role for our Lambda function.
- Create a Lambda function in the AWS Management Console.
- Write the Node.js code for our Lambda function.
- Test our Lambda function.
- Create an Amazon API Gateway REST API to trigger our Lambda function.
- Deploy our application.
Let’s go through each of these steps in detail.
Step 1: Set Up an AWS Account and Create an IAM Role
To get started with AWS Lambda, we first need to create an AWS account if we don’t already have one. Once we have an AWS account, we need to create an IAM role that our Lambda function will use to access other AWS services like Amazon S3 and Amazon DynamoDB.
To create an IAM role, we can follow these steps:
- Go to the AWS Management Console and navigate to the IAM service.
- Click on “Roles” in the left-hand menu and then click the “Create role” button.
- Select “Lambda” as the AWS service that will use this role and click “Next: Permissions.”
- Select the permissions that our Lambda function will need to access other AWS services. For example, if our Lambda function needs to access an Amazon S3 bucket, we need to grant it permission to read and write objects in that bucket.
- Click “Next: Tags” and then “Next: Review” to review our role settings.
- Give our role a name and click “Create role” to create the IAM role.
Step 2: Create a Lambda Function in the AWS Management Console
Now that we have an IAM role set up, we can create a Lambda function in the AWS Management Console. To create a Lambda function, we can follow these steps:
- Go to the AWS Management Console and navigate to the Lambda service.
- Click on the “Create function” button.
- Select “Author from scratch” and enter a name for our function.
- Choose “Node.js” as the runtime and select the IAM role we created in Step 1 as the execution role.
- Click “Create function” to create the Lambda function.
Step 3: Write the Node.js Code for Our Lambda Function
Now that we have a Lambda function set up, we need to write the Node.js code that our function will execute. In this example, we will create a simple function that retrieves data from an Amazon DynamoDB table and returns it as a JSON object.
Here is an example of the Node.js code we will use:
const AWS = require('aws-sdk');
exports.handler = async (event) => {
const dynamodb = new AWS.DynamoDB({apiVersion: '2012-08-10'});
const params = {
TableName: 'my-dynamodb-table',
Key: {
'id': {S: '1'}
}
};
try {
const data = await dynamodb.getItem(params).promise();
const response = {
statusCode: 200,
body: JSON.stringify(data.Item)
};
return response;
} catch (err) {
console.log(err);
return err;
}
};
In this code, we first import the AWS SDK for Node.js and then define our Lambda function handler. Our handler takes an event parameter, which is passed in by AWS Lambda, and uses it to retrieve data from an Amazon DynamoDB table. We then format the data as a JSON object and return it as the response from our Lambda function.
Step 4: Test Our Lambda Function
Before we deploy our application, we should test our Lambda function to make sure it is working correctly. We can test our function in the AWS Management Console by clicking on the “Test” button.
In the test panel, we can configure a test event to pass to our function. For example, we could create a test event that simulates an HTTP request by passing in a JSON object with an “HTTP method” property set to “GET”.
Once we have configured our test event, we can click the “Test” button to execute our Lambda function. If everything is working correctly, we should see a successful response from our function.
Step 5: Create an Amazon API Gateway REST API to Trigger Our Lambda Function
Now that we have a working Lambda function, we can create an Amazon API Gateway REST API to trigger our function. Amazon API Gateway is a fully managed service that makes it easy to create, publish, and manage APIs.
To create an Amazon API Gateway REST API, we can follow these steps:
- Go to the AWS Management Console and navigate to the API Gateway service.
- Click on “Create API” and select “REST API.”
- Choose “New API” and give our API a name.
- Click “Create API” to create the API.
Now that we have created our API, we need to create a resource and a method to trigger our Lambda function. To do this, we can follow these steps:
- Click on the “Create Resource” button and give our resource a name.
- Click on our new resource and then click on the “Create Method” button.
- Select the HTTP method we want to use (e.g., GET) and choose “Lambda Function” as the integration type.
- Choose our Lambda function from the dropdown menu and click “Save.”
Step 6: Deploy Our Application
Finally, we need to deploy our application so that it is accessible over the internet. To deploy our application, we can follow these steps:
- Click on the “Actions” dropdown menu and choose “Deploy API.”
- Choose the deployment stage we want to deploy to (e.g., “prod”) and click “Deploy.”
- Copy the URL for our API endpoint, which should be displayed in the “Invoke URL” section.
Now that our application is deployed, we can test it by sending an HTTP request to the API endpoint we just created. For example, if our API endpoint is “https://abc123.execute-api.us-east-1.amazonaws.com/prod“, we could send a GET request to “https://abc123.execute-api.us-east-1.amazonaws.com/prod/my-resource” to trigger our Lambda function and retrieve data from our Amazon DynamoDB table.
Conclusion
In this blog post, we have explored how to create a serverless application with Node.js and AWS Lambda. We started by understanding the basics of serverless computing and AWS Lambda, and then we walked through the process of building a serverless application that uses AWS Lambda and other AWS services.
By leveraging the power of serverless computing, we can build applications that are more scalable, more reliable, and easier to manage than traditional server-based applications. With AWS Lambda and Node.js, we have a powerful toolkit for building serverless applications that can respond to a wide range of events and handle complex business logic.
If you are new to serverless computing, we encourage you to try out some of the examples and tutorials provided by AWS and other cloud providers. With a little practice, you will soon be able to build complex and powerful applications that take advantage of the full potential of serverless computing.
Responses