How to Optimize Your Code Using Data Structures: Tips and Tricks
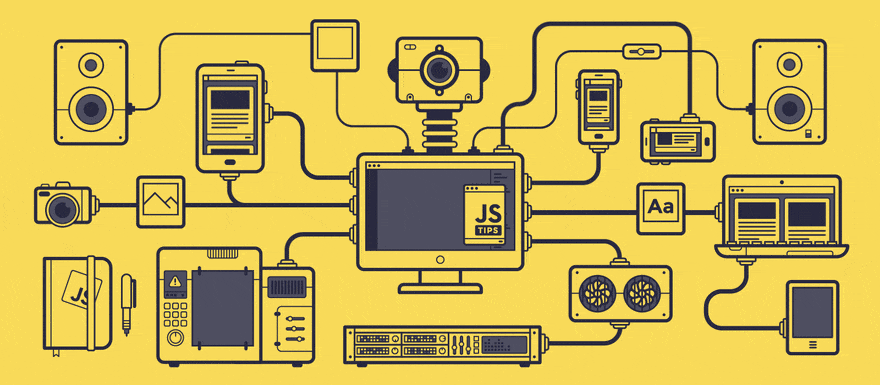
Introduction
When it comes to software development, writing efficient code is crucial for good performance. In this blog post, we’ll explore how data structures can be used to optimize code and improve its efficiency. Specifically, we’ll be using JavaScript as our programming language of choice.
Data Structures in JavaScript
Before we dive into the specifics of how to optimize code using data structures, it’s important to have a basic understanding of data structures in JavaScript. Data structures are essentially ways of organizing and storing data in a program. Some common data structures in JavaScript include arrays, objects, maps, and sets.
Arrays
Arrays are a commonly used data structure in JavaScript. They can be used to store collections of data, such as lists of numbers, strings, or objects. Arrays are typically accessed using an index, with the first element in the array having an index of 0.
For example, consider the following array:
const numbers = [1, 2, 3, 4, 5];
To access the third element in the array, we would use the following code:
const thirdElement = numbers[2];
Objects
Objects are another commonly used data structure in JavaScript. They are used to store collections of key-value pairs. For example, consider the following object:
const person = {
name: 'John',
age: 30,
email: 'john@example.com'
};
To access the age property of this object, we would use the following code:
const age = person.age;
Maps
Maps are a newer addition to JavaScript, added in ES6. They are similar to objects in that they store key-value pairs, but they have some additional features that make them more flexible. Maps can use any data type as a key, whereas objects are limited to using strings or symbols as keys.
To create a map in JavaScript, we use the following code:
const map = new Map();
We can then add key-value pairs to the map using the set()
method:
map.set('key1', 'value1');
map.set('key2', 'value2');
To retrieve a value from a map, we use the get()
method:
const value = map.get('key1');
Sets
Sets are another newer addition to JavaScript, also added in ES6. They are used to store collections of unique values. For example, consider the following set:
const set = new Set([1, 2, 3]);
To add a value to a set, we use the add()
method:
set.add(4);
To check if a value is in a set, we use the has()
method:
const hasValue = set.has(3);
Now that we have a basic understanding of data structures in JavaScript, let’s explore how they can be used to optimize code.
Optimizing Code with Data Structures
- Using Arrays for Iteration
One of the most common use cases for arrays is iterating over their elements. However, it’s important to use the correct iteration method for the task at hand. The for
loop is the most basic way to iterate over an array, but it can be slow for large arrays. A more efficient way to iterate over an array is to use the forEach()
method:
const numbers = [1, 2, 3, 4, 5];
numbers.forEach((number) => {
console.log(number);
});
The forEach()
method is faster than a for
loop because it doesn’t require
the overhead of initializing a loop counter and checking the condition on every iteration. Additionally, it can be used with arrow functions, making it more concise and easier to read.
- Using Objects for Quick Access
Objects can be a great choice when you need to quickly access specific values by their keys. This is because accessing a value in an object by its key is typically faster than searching through an array for a specific value. For example, consider the following object:
const person = {
name: 'John',
age: 30,
email: 'john@example.com'
};
If we wanted to retrieve the person’s age, we could simply access it directly using the age
key:
const age = person.age;
This is faster than searching through an array for a person object with a specific name or email address.
- Using Maps for Flexibility
Maps can be a good choice when you need more flexibility than what an object provides. For example, maps can use any data type as a key, whereas objects are limited to using strings or symbols as keys. Additionally, maps can be iterated over in a specific order, whereas objects have no guaranteed order.
One example use case for maps is caching expensive calculations. For example, consider the following function:
function fibonacci(n) {
if (n <= 1) {
return n;
}
return fibonacci(n - 1) + fibonacci(n - 2);
}
This function calculates the nth Fibonacci number recursively. However, it can be very slow for large values of n. To speed up this function, we could use a map to cache the results of previous calculations:
const fibCache = new Map();
function fibonacci(n) {
if (n <= 1) {
return n;
}
if (fibCache.has(n)) {
return fibCache.get(n);
}
const result = fibonacci(n - 1) + fibonacci(n - 2);
fibCache.set(n, result);
return result;
}
In this example, we create a new map called fibCache
to store the results of previous calculations. When the fibonacci()
function is called with a value of n, it first checks if the result is already cached in the fibCache
map. If it is, the cached value is returned instead of recalculating the result. If the result is not cached, the function calculates it recursively as before, and then caches the result in the map for future use.
- Using Sets for Unique Values
Sets can be a good choice when you need to store a collection of unique values. This is because sets automatically remove duplicate values. For example, consider the following array:
const numbers = [1, 2, 3, 3, 4, 5, 5, 5];
If we wanted to remove the duplicate values from this array, we could use a set:
const uniqueNumbers = new Set(numbers);
The uniqueNumbers
set would then contain the unique values from the numbers
array.
- Choosing the Right Data Structure for the Task
When optimizing code using data structures, it’s important to choose the right data structure for the task at hand. Each data structure has its own strengths and weaknesses, and using the wrong data structure can actually make code less efficient. For example, using an object to store a large collection of values that need to be accessed in a specific order can be less efficient than using an array or a map.
Conclusion
In this blog post, we explored how data structures can be used to optimize code and improve its efficiency. We covered some common data structures in JavaScript, including arrays, objects, maps, and sets. We then explored some tips and tricks for optimizing code using these data structures, such as using arrays for iteration, using objects for quick access, using maps for flexibility, using sets for unique values, and choosing the right data structure for the task.
By using data structures effectively, you can significantly improve the performance of your code. However, it’s important to keep in mind that optimization should be done carefully and only when necessary. Premature optimization can lead to code that is harder to read and maintain, and may even reduce performance in some cases.
Overall, data structures are an important tool in any developer’s toolkit, and understanding how to use them effectively can make a big difference in the efficiency and performance of your code.
Responses