Unlocking the Power of Node.js Native Transform API: A Guide to Making Any Object Observable with Raw Node.js
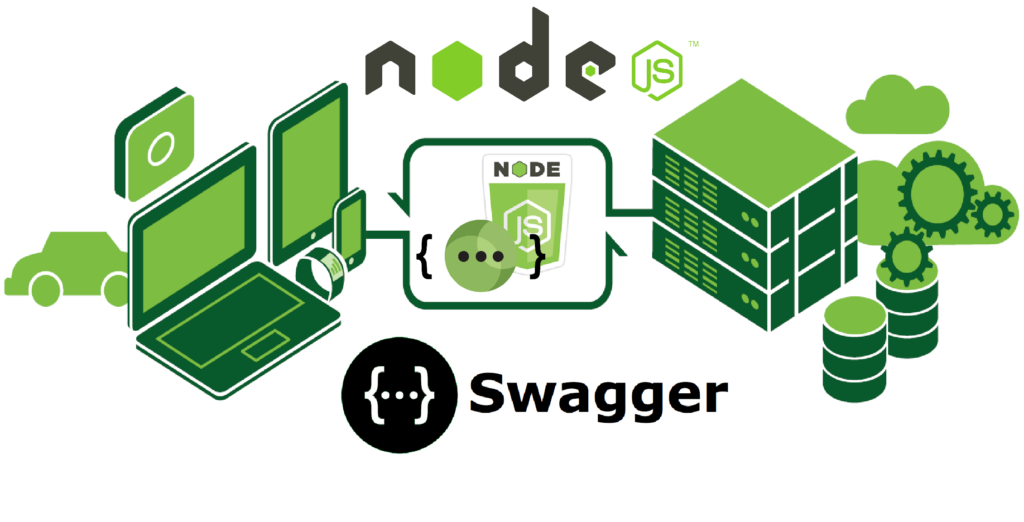
Node.js is a popular platform for building scalable and high-performance web applications. It provides a powerful set of features for building server-side applications, such as event-driven programming, non-blocking I/O, and real-time communication. One of the lesser-known features of Node.js is the NativeTransform API, which allows you to make any object observable.
In this post, we’ll explore the NativeTransform API and show you how to use it to make any object observable. We’ll cover the basics of observables, explain how the NativeTransform API works, and provide some examples to get you started.
What Are Observables?
An observable is a powerful programming concept that allows you to monitor changes to an object and respond to those changes in real-time. It’s commonly used in reactive programming, where you want to create applications that respond to user actions or data changes in real-time.
In Node.js, observables are implemented using the NativeTransform API. This API provides a way to create an observable stream of data from any object, allowing you to monitor changes to that object and react accordingly.
How the NativeTransform API Works
The NativeTransform API is a set of classes and functions that allow you to create a transform stream. A transform stream is a stream that can be both read from and written to, and can modify or transform the data as it passes through the stream.
To create an observable using the NativeTransform API, you first need to create a transform stream that can be written to. You can then use the write
method of the transform stream to write data to the stream, and the read
method to read data from the stream.
When data is written to the stream, it’s passed through a transform function that can modify or transform the data. This function is called the transform callback, and it’s where you can implement your observable logic.
In the transform callback, you can monitor changes to the data and emit events when those changes occur. You can also modify the data before it’s passed through the stream, allowing you to transform the data in real-time.
Examples
Let’s take a look at some examples of how you can use the NativeTransform API to create observables.
Example 1: Monitoring Changes to an Object
In this example, we’ll create an observable that monitors changes to an object. We’ll use the NativeTransform API to create a transform stream that can be written to, and we’ll implement a transform callback that monitors changes to the data and emits events when those changes occur.
const { Transform } = require('stream');
class Observable extends Transform {
constructor(object) {
super({ objectMode: true });
this.object = object;
}
_transform(data, encoding, callback) {
this.object = { ...this.object, ...data };
this.emit('change', this.object);
callback(null, this.object);
}
}
const obj = { foo: 'bar' };
const observable = new Observable(obj);
observable.on('change', (data) => {
console.log('Object changed', data);
});
observable.write({ baz: 'qux' });
// Output: Object changed { foo: 'bar', baz: 'qux' }
In this example, we created a new Observable
class that extends the Transform
class from the stream
module. We passed an object to the constructor, which is the object we want to monitor.
In the _transform
method, we merged the incoming data with the existing object and emitted a change
event with the updated object. We also called the callback
function to indicate that we’ve finished processing the data.
We then created a new instance of the Observable
class and passed our object to it. We attached an event listener to the change
event and logged the updated object when the event is emitted.
Finally, we wrote some new data to the observable using the write
method. When the data is written to the observable, the _transform
method is called, and the changes are detected and emitted as a change
event.
Example 2: Transforming Data in Real-Time
In this example, we’ll create an observable that transforms data in real-time. We’ll use the NativeTransform API to create a transform stream that can be written to, and we’ll implement a transform callback that doubles any number that’s written to the stream.
In this example, we created a new Observable
class that extends the Transform
class from the stream
module. In the _transform
method, we checked if the incoming data is a number. If it is, we doubled the number and emitted a double
event with the doubled number. If it’s not a number, we simply pass the data through unchanged.
We then created a new instance of the Observable
class and attached an event listener to the double
event. When the event is emitted, we log the doubled number to the console.
Finally, we wrote the number 2 to the observable using the write
method. The _transform
method detected that the data is a number and doubled it, emitting a double
event with the value 4.
const { Transform } = require('stream');
class Observable extends Transform {
constructor() {
super({ objectMode: true });
}
_transform(data, encoding, callback) {
if (typeof data === 'number') {
const doubled = data * 2;
this.emit('double', doubled);
callback(null, doubled);
} else {
callback(null, data);
}
}
}
const observable = new Observable();
observable.on('double', (data) => {
console.log('Doubled', data);
});
observable.write(2);
// Output: Doubled 4
"use strict";
/**
*
* @module ObservableTransform
* @kind class
*
* @extends Transform
* @requires Transform
* @requires get
* @requires request
* @requires parse
* @classdesc ObservableTransform class
*/
class ObservableTransform extends require("stream").Transform {
constructor(...arrayOfObjects) {
super({ objectMode: true, encoding: "utf-8", autoDestroy: true });
arrayOfObjects.forEach(option => {
if(Object.keys(option).length > 0){
Object.keys(option).forEach((key) => { if(!this[key]) this[key] = option[key];})
}
});
if(this.observers) this.observers = new Set();
//Set the maximum number of listeners to infinity
this.setMaxListeners(Infinity);
}
}
The code defines a class named ObservableTransform
that extends the built-in Transform
class of Node.js. The Transform
class is a stream that can transform input data and produce output data.
The ObservableTransform
class has a constructor that takes an array of objects as an argument. The constructor first calls the super
method with an options object that sets the stream to operate in object mode, use UTF-8 encoding, and auto-destroy itself when it is no longer needed.
The constructor then loops over the array of objects and assigns their properties to the instance of the ObservableTransform
class. Specifically, for each option object, it checks if the object has any properties and then assigns those properties to the instance if they do not already exist.
Finally, the constructor sets the maximum number of listeners to infinity on the instance of the ObservableTransform
class and initializes a Set
object named observers
if the class does not already have it defined.
Conclusion
The NativeTransform API in Node.js provides a powerful way to make any object observable. By creating a transform stream and implementing a transform callback, you can monitor changes to an object and react in real-time.
In this post, we’ve covered the basics of observables, explained how the NativeTransform API works, and provided some examples to get you started. With this knowledge, you can start using observables in your Node.js applications to create reactive and responsive user experiences.
Responses