Implementing Graph Algorithms in Your Project: A Step-by-Step Guide
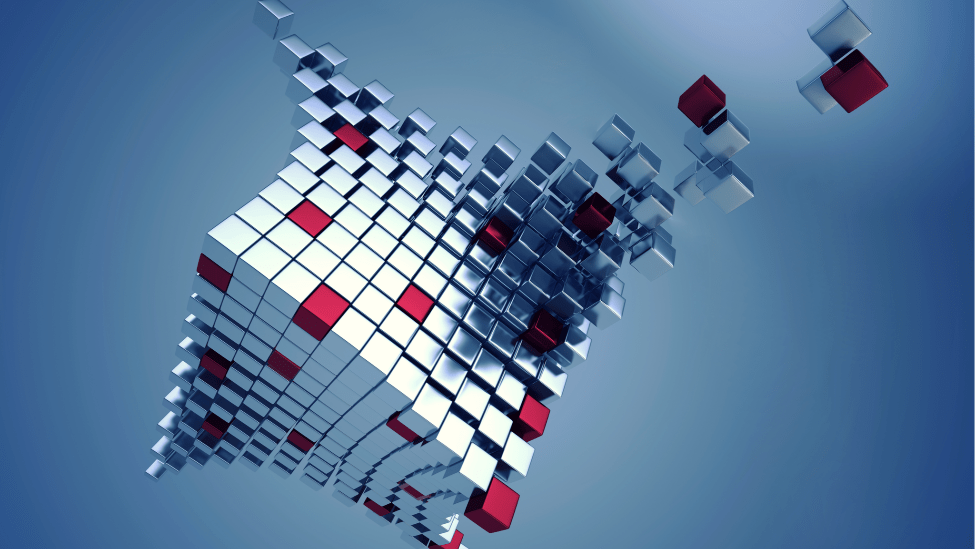
In this blog post, I’ll be discussing how you can implement graph algorithms in your project using Javascript. I’ll provide you with a step-by-step guide, so that you can easily follow along.
Before we get started, let’s first understand what graphs and graph algorithms are.
What are Graphs and Graph Algorithms?
In computer science, a graph is a data structure that consists of a set of vertices (also called nodes or points) and a set of edges (also called arcs or lines) that connect pairs of vertices. Graphs are used to represent many real-world applications, such as social networks, transportation networks, and electrical circuits.
A graph algorithm is an algorithm that operates on a graph, either to find certain properties of the graph, or to solve a specific problem on the graph. There are many different graph algorithms, such as traversal algorithms (e.g. depth-first search and breadth-first search), shortest path algorithms (e.g. Dijkstra’s algorithm and Bellman-Ford algorithm), and spanning tree algorithms (e.g. Kruskal’s algorithm and Prim’s algorithm).
Now that we have a basic understanding of graphs and graph algorithms, let’s move on to implementing them in Javascript.
Step 1: Create a Graph Data Structure
The first step in implementing graph algorithms in your project is to create a graph data structure. There are many ways to represent a graph in Javascript, but one of the most common ways is to use an adjacency list.
An adjacency list is a collection of arrays, where each array represents a vertex in the graph, and the elements of the array represent the vertices that are adjacent to that vertex. Here’s an example of an adjacency list for a simple graph:
const graph = [
[1, 2], // vertex 0 is adjacent to vertices 1 and 2
[0, 2], // vertex 1 is adjacent to vertices 0 and 2
[0, 1], // vertex 2 is adjacent to vertices 0 and 1
];
In this example, the graph has three vertices (0, 1, and 2), and each vertex is adjacent to two other vertices.
Step 2: Implement Graph Algorithms
Once you have created a graph data structure, you can start implementing graph algorithms. Let’s start with a simple algorithm: depth-first search (DFS).
DFS is a traversal algorithm that visits all the vertices in a graph in a depth-first manner. Here’s how you can implement DFS in Javascript using recursion:
function dfs(graph, start, visited = new Set()) {
visited.add(start);
for (const neighbor of graph[start]) {
if (!visited.has(neighbor)) {
dfs(graph, neighbor, visited);
}
}
return visited;
}
In this implementation, graph
is the adjacency list representing the graph, start
is the vertex to start the traversal from, and visited
is a set of visited vertices (initialized as an empty set).
The function starts by adding the start
vertex to the visited
set. It then loops through all the neighbors of start
, and recursively calls dfs
on each unvisited neighbor. Finally, the function returns the visited
set.
Let’s test the dfs
function on the example graph we created earlier:
const graph = [
[1, 2], // vertex 0 is adjacent to vertices 1 and 2
[0, 2], // vertex 1 is adjacent to vertices 0 and 2
[0, 1], // vertex 2 is adjacent to vertices 0 and 1
];
const visited = dfs(graph, 0);
console.log(visited); // Set {0, 1, 2}
In this example, we start the traversal from vertex 0, and the visited
set contains all three vertices (0, 1, and 2).
Next, let’s implement another traversal algorithm: breadth-first search (BFS).
BFS is a traversal algorithm that visits all the vertices in a graph in a breadth-first manner. Here’s how you can implement BFS in Javascript using a queue:
function bfs(graph, start) {
const queue = [start];
const visited = new Set([start]);
while (queue.length > 0) {
const current = queue.shift();
for (const neighbor of graph[current]) {
if (!visited.has(neighbor)) {
visited.add(neighbor);
queue.push(neighbor);
}
}
}
return visited;
}
In this implementation, graph
is the adjacency list representing the graph, and start
is the vertex to start the traversal from.
The function starts by initializing a queue with the start
vertex and a visited set with the start
vertex. It then enters a while loop that continues as long as the queue is not empty. In each iteration of the loop, the function dequeues the first vertex in the queue and adds its unvisited neighbors to the queue and visited set.
Let’s test the bfs
function on the example graph we created earlier:
const graph = [
[1, 2], // vertex 0 is adjacent to vertices 1 and 2
[0, 2], // vertex 1 is adjacent to vertices 0 and 2
[0, 1], // vertex 2 is adjacent to vertices 0 and 1
];
const visited = bfs(graph, 0);
console.log(visited); // Set {0, 1, 2}
In this example, we start the traversal from vertex 0, and the visited
set contains all three vertices (0, 1, and 2).
Finally, let’s implement Dijkstra’s algorithm, which is a shortest path algorithm that finds the shortest path between a starting vertex and all other vertices in the graph.
Dijkstra’s algorithm works by maintaining a priority queue of vertices to visit, with the vertex with the smallest distance from the starting vertex at the front of the queue. Here’s how you can implement Dijkstra’s algorithm in Javascript using a priority queue:
function dijkstra(graph, start) {
const distances = Array(graph.length).fill(Infinity);
distances[start] = 0;
const pq = new PriorityQueue();
pq.enqueue(start, 0);
while (!pq.isEmpty()) {
const current = pq.dequeue().element;
for (const neighbor of graph[current]) {
const distance = distances[current] + 1;
if (distance < distances[neighbor]) {
distances[neighbor] = distance;
pq.enqueue(neighbor, distance);
}
}
}
return distances;
}
In this implementation, graph
is the adjacency list representing the graph, and start
is the vertex to start the shortest path search from.
In this implementation, graph
is the adjacency list representing the graph, and start
is the vertex to start the shortest path search from.
The function starts by initializing an array distances
of length graph.length
with all elements set to Infinity
, except for the start
vertex, which is set to 0. It also initializes a priority queue pq
with the start
vertex and its distance from the starting vertex (which is 0).
The function enters a while loop that continues as long as the priority queue is not empty. In each iteration of the loop, the function dequeues the vertex with the smallest distance from the starting vertex, and updates the distances of its unvisited neighbors. If the distance to a neighbor is smaller than its current distance, the neighbor’s distance is updated, and the neighbor is added to the priority queue.
Let’s test the dijkstra
function on the example graph we created earlier:
const graph = [
[1, 2], // vertex 0 is adjacent to vertices 1 and 2
[0, 2], // vertex 1 is adjacent to vertices 0 and 2
[0, 1], // vertex 2 is adjacent to vertices 0 and 1
];
const distances = dijkstra(graph, 0);
console.log(distances); // [0, 1, 1]
In this example, we start the shortest path search from vertex 0, and the distances
array contains the shortest distance from vertex 0 to all other vertices (0 to itself, 1 to vertex 1 and 1 to vertex 2).
Step 3: Integrate Graph Algorithms in Your Project
Now that we have implemented graph algorithms in Javascript, we can integrate them into our project. Depending on the requirements of your project, you may want to use one or more of the algorithms we have implemented.
For example, if your project involves social networking, you may want to use DFS to find all the friends of a user, or BFS to find the shortest path between two users. If your project involves transportation networks, you may want to use Dijkstra’s algorithm to find the shortest path between two locations.
To integrate the graph algorithms in your project, you can simply copy and paste the implementations we have provided into your project code, and call them with the appropriate parameters.
Conclusion
In this blog post, we have discussed how you can implement graph algorithms in your project using Javascript. We have provided you with a step-by-step guide and implemented three algorithms: depth-first search (DFS), breadth-first search (BFS), and Dijkstra’s algorithm.
By following the steps we have provided, you can easily integrate graph algorithms into your project and use them to solve a wide range of problems. Whether your project involves social networking, transportation networks, or any other type of graph, the algorithms we have implemented will help you navigate and analyze your data efficiently.
Responses