Sorting Algorithms Explained: How to Choose the Right One for Your Project
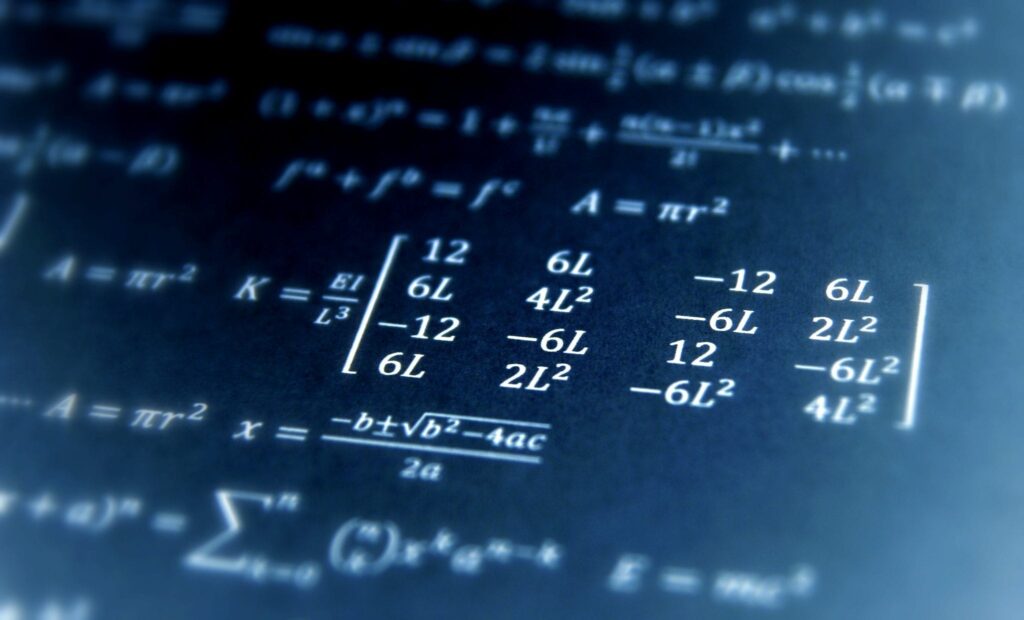
Sorting algorithms are essential tools for computer science and programming. They enable us to organize data in an efficient and organized way, making it easier to find and manipulate information. In this post, I’ll explain what sorting algorithms are, the different types of sorting algorithms, and how to choose the right one for your project.
What are Sorting Algorithms?
Sorting algorithms are methods or procedures used to arrange a list of elements in a specific order. These algorithms can sort elements in either ascending or descending order. For example, sorting a list of numbers in ascending order would result in a list with the smallest number at the beginning and the largest number at the end.
Sorting algorithms are essential for many different applications, such as databases, search engines, and scientific computing. They enable faster access and processing of information, making it easier to find and analyze data.
Types of Sorting Algorithms
There are several types of sorting algorithms, each with its strengths and weaknesses. The most commonly used sorting algorithms include:
- Bubble Sort
Bubble sort is one of the simplest sorting algorithms. It works by repeatedly swapping adjacent elements if they are in the wrong order. The algorithm continues swapping elements until the entire list is sorted. Bubble sort has a time complexity of O(n^2), which means that it is not efficient for large lists.
- Selection Sort
Selection sort is another simple sorting algorithm that works by repeatedly finding the minimum element in the list and moving it to the front. The algorithm continues to find the minimum element and move it to the front until the entire list is sorted. Selection sort also has a time complexity of O(n^2).
- Insertion Sort
Insertion sort is another simple sorting algorithm that works by sorting elements one at a time. It starts by comparing the first two elements and swapping them if they are in the wrong order. The algorithm continues to compare the current element with the previous one until the entire list is sorted. Insertion sort has a time complexity of O(n^2).
- Quick Sort
Quick sort is a more efficient sorting algorithm that works by selecting a pivot element from the list and partitioning the list into two sub-lists. The elements in the left sub-list are smaller than the pivot, while the elements in the right sub-list are larger than the pivot. The algorithm then recursively sorts the two sub-lists. Quick sort has a time complexity of O(nlogn), making it more efficient than the previous algorithms.
- Merge Sort
Merge sort is another efficient sorting algorithm that works by dividing the list into two halves, sorting each half, and then merging the two halves back together. The algorithm uses a divide-and-conquer approach to sort the list. Merge sort also has a time complexity of O(nlogn).
- Heap Sort
Heap sort is a sorting algorithm that works by building a binary heap from the list and then repeatedly removing the root element and placing it at the end of the sorted list. The algorithm continues to remove the root element until the entire list is sorted. Heap sort has a time complexity of O(nlogn).
Choosing the Right Sorting Algorithm
When choosing a sorting algorithm, it’s essential to consider the size of the list, the type of data being sorted, and the specific requirements of the project. Different sorting algorithms have different strengths and weaknesses, and choosing the right one can significantly impact the performance and efficiency of your project.
For example, if you’re working with small lists, simple sorting algorithms like the bubble sort, selection sort, or insertion sort may be sufficient. However, for larger lists, more efficient algorithms like quick sort, merge sort, or heap sort may be necessary.
It’s also essential to consider
the data type being sorted. Some sorting algorithms may work better with certain data types than others. For example, if you’re sorting strings, a sorting algorithm that compares strings character by character, like merge sort, may be more efficient than a sorting algorithm that converts strings to integers and sorts them numerically.
Another factor to consider is the stability of the sorting algorithm. A stable sorting algorithm maintains the relative order of equal elements in the list. For example, if you’re sorting a list of students by their grade point average and two students have the same GPA, a stable sorting algorithm will maintain the order of those students based on their original order in the list. On the other hand, an unstable sorting algorithm may change the order of equal elements. In some cases, stability may be essential for the project’s requirements.
Finally, it’s essential to consider the space complexity of the sorting algorithm. Space complexity refers to the amount of memory required to execute the algorithm. Some sorting algorithms require additional memory to store temporary variables or to perform the sorting operation. This additional memory can be a concern if the project has limited memory resources.
Conclusion
Sorting algorithms are essential tools for computer science and programming. They enable us to organize data efficiently, making it easier to find and manipulate information. There are several types of sorting algorithms, each with its strengths and weaknesses. When choosing a sorting algorithm, it’s important to consider the size of the list, the type of data being sorted, the stability of the algorithm, and the space complexity of the algorithm. By carefully considering these factors, you can choose the right sorting algorithm for your project and optimize its performance and efficiency.
In summary, here are some guidelines for choosing the right sorting algorithm:
- Consider the size of the list: Simple sorting algorithms like bubble sort or selection sort may be sufficient for small lists, while more efficient algorithms like quick sort, merge sort, or heap sort may be necessary for larger lists.
- Consider the type of data being sorted: Different sorting algorithms may work better with certain data types than others. For example, a sorting algorithm that compares strings character by character may be more efficient for sorting strings than a sorting algorithm that converts strings to integers and sorts them numerically.
- Consider the stability of the algorithm: If maintaining the relative order of equal elements is important, choose a stable sorting algorithm.
- Consider the space complexity of the algorithm: If the project has limited memory resources, choose a sorting algorithm with a low space complexity.
It’s also important to note that many programming languages come with built-in sorting functions that use optimized sorting algorithms. These functions are often optimized for the language and platform they’re running on and can be faster and more efficient than implementing a sorting algorithm from scratch.
After all, choosing the right sorting algorithm is an important decision that can significantly impact the performance and efficiency of your project. By considering the size of the list, the type of data being sorted, the stability of the algorithm, and the space complexity of the algorithm, you can choose the right sorting algorithm for your project and optimize its performance and efficiency.
Responses